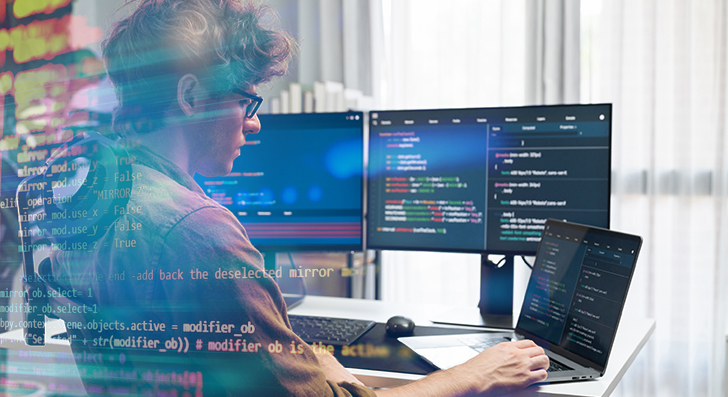
Scalability usually means your application can handle expansion—far more consumers, much more details, plus more website traffic—with no breaking. As being a developer, setting up with scalability in mind saves time and stress afterwards. Right here’s a transparent and functional manual to help you start out by Gustavo Woltmann.
Design and style for Scalability from the Start
Scalability is just not anything you bolt on later—it ought to be element within your prepare from the beginning. A lot of apps fail if they expand speedy since the first design and style can’t handle the extra load. To be a developer, you'll want to Believe early regarding how your method will behave stressed.
Start off by creating your architecture to get versatile. Stay away from monolithic codebases exactly where every thing is tightly related. Alternatively, use modular design and style or microservices. These styles split your application into more compact, independent areas. Each and every module or service can scale By itself without having influencing The full method.
Also, think about your database from working day 1. Will it will need to deal with 1,000,000 buyers or simply just a hundred? Choose the proper style—relational or NoSQL—according to how your information will increase. System for sharding, indexing, and backups early, Even when you don’t need them nonetheless.
An additional critical place is to avoid hardcoding assumptions. Don’t write code that only is effective less than present-day conditions. Consider what would materialize In the event your person foundation doubled tomorrow. Would your app crash? Would the database slow down?
Use style patterns that aid scaling, like concept queues or event-pushed programs. These support your app handle far more requests without having obtaining overloaded.
When you Make with scalability in mind, you are not just getting ready for fulfillment—you are reducing long run problems. A properly-prepared technique is less complicated to take care of, adapt, and increase. It’s much better to get ready early than to rebuild later.
Use the appropriate Databases
Selecting the ideal database can be a key A part of constructing scalable purposes. Not all databases are developed the same, and using the Incorrect one can slow you down or maybe cause failures as your app grows.
Start by knowing your knowledge. Could it be remarkably structured, like rows inside of a table? If Of course, a relational database like PostgreSQL or MySQL is an efficient healthy. These are potent with relationships, transactions, and regularity. In addition they aid scaling techniques like examine replicas, indexing, and partitioning to take care of additional targeted visitors and facts.
If the information is more adaptable—like consumer activity logs, product catalogs, or paperwork—contemplate a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at managing massive volumes of unstructured or semi-structured details and might scale horizontally a lot more effortlessly.
Also, consider your examine and write styles. Do you think you're carrying out lots of reads with less writes? Use caching and browse replicas. Are you currently managing a hefty produce load? Consider databases which will handle substantial write throughput, and even celebration-based data storage programs like Apache Kafka (for non permanent information streams).
It’s also sensible to Assume in advance. You might not need Superior scaling capabilities now, but deciding on a databases that supports them suggests you received’t have to have to switch later.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your data based on your access patterns. And always watch databases effectiveness while you increase.
In a nutshell, the right databases will depend on your application’s construction, velocity requires, And the way you expect it to grow. Choose time to choose wisely—it’ll help you save plenty of difficulty afterwards.
Enhance Code and Queries
Quick code is essential to scalability. As your application grows, just about every tiny hold off adds up. Improperly prepared code or unoptimized queries can decelerate efficiency and overload your procedure. That’s why it’s crucial that you build successful logic from the start.
Begin by producing clean, uncomplicated code. Steer clear of repeating logic and remove just about anything pointless. Don’t select the most complex Remedy if a straightforward 1 performs. Maintain your features small, concentrated, and straightforward to test. Use profiling resources to find bottlenecks—areas the place your code usually takes too extended to run or uses an excessive amount of memory.
Up coming, have a look at your database queries. These typically sluggish things down greater than the code itself. Make sure each question only asks for the information you actually need. Avoid Find *, which fetches all the things, and as a substitute decide on specific fields. Use indexes to hurry up lookups. And keep away from carrying out a lot of joins, In particular across substantial tables.
In case you discover a similar knowledge becoming asked for repeatedly, use caching. Keep the results temporarily applying resources like Redis or Memcached this means you don’t really need to repeat high priced operations.
Also, batch your database operations after you can. As opposed to updating a row one after the other, update them in teams. This cuts down on overhead and would make your application a lot more successful.
Make sure to test with huge datasets. Code and queries that perform great with 100 records may well crash whenever they have to manage one million.
In brief, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when necessary. These methods assist your application stay smooth and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to deal with much more customers and much more visitors. If every thing goes via one server, it can speedily become a bottleneck. That’s where load balancing and caching are available. These two tools assistance keep your application quickly, stable, and scalable.
Load balancing spreads incoming website traffic across multiple servers. In lieu of 1 server accomplishing every one of the function, the load balancer routes people to distinct servers based on availability. What this means is no solitary server receives overloaded. If a single server goes down, the load balancer can mail traffic to the Some others. Tools like Nginx, HAProxy, or cloud-based mostly remedies from AWS and Google Cloud make this straightforward to create.
Caching is about storing details temporarily so it may be reused promptly. When customers request the identical facts all over again—like an item page or even a profile—you don’t ought to fetch it within the database anytime. You could provide it from your cache.
There's two widespread kinds of caching:
one. Server-side caching (like Redis or Memcached) suppliers info in memory for fast entry.
2. Client-facet caching (like browser caching or CDN caching) shops static data files close to the person.
Caching minimizes databases load, improves pace, and makes your app extra productive.
Use caching for things which don’t modify normally. And often make certain your cache is up-to-date when data does adjust.
In short, load balancing and caching are straightforward but impressive equipment. Alongside one another, they help your app cope with more consumers, continue to be fast, and Recuperate from problems. If you intend to improve, you'll need equally.
Use Cloud and Container Applications
To build scalable programs, you may need applications that let your app expand quickly. That’s where cloud platforms and containers come in. They provide you overall flexibility, lower setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and providers as you may need them. You don’t should invest in components or guess future capacity. When visitors raises, you'll be able to incorporate far more means with just a few clicks or automatically using auto-scaling. When traffic drops, you are able to scale down to save money.
These platforms also offer services like managed databases, storage, load balancing, and security tools. You are able to concentrate on building your application in lieu of running infrastructure.
Containers are A further critical tool. A container offers your application and almost everything it should run—code, libraries, settings—into one device. This causes it to be straightforward to move your application amongst environments, out of your laptop into the cloud, devoid of surprises. Docker is the most well-liked Instrument for this.
Whenever your app takes advantage of a number of containers, website equipment like Kubernetes enable you to handle them. Kubernetes handles deployment, scaling, and Restoration. If one particular element of your application crashes, it restarts it instantly.
Containers also make it straightforward to independent parts of your application into providers. You can update or scale sections independently, and that is great for performance and dependability.
In short, working with cloud and container resources suggests you'll be able to scale speedy, deploy simply, and recover speedily when problems transpire. If you would like your application to develop devoid of limits, start off using these applications early. They preserve time, cut down threat, and assist you stay centered on building, not repairing.
Watch Every thing
When you don’t monitor your application, you gained’t know when matters go Incorrect. Checking can help the thing is how your app is doing, location problems early, and make superior decisions as your app grows. It’s a crucial Component of building scalable methods.
Start off by monitoring essential metrics like CPU usage, memory, disk Area, and response time. These inform you how your servers and expert services are doing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this knowledge.
Don’t just observe your servers—monitor your app too. Regulate how much time it's going to take for users to load pages, how often errors occur, and exactly where they take place. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Create alerts for crucial difficulties. By way of example, When your response time goes over a limit or perhaps a service goes down, you should get notified instantly. This will help you correct troubles quickly, usually prior to users even see.
Checking can be beneficial whenever you make changes. For those who deploy a different element and see a spike in errors or slowdowns, you could roll it back again just before it leads to real problems.
As your app grows, visitors and details raise. Without the need of monitoring, you’ll miss indications of problems until it’s much too late. But with the best tools set up, you remain on top of things.
In brief, checking aids you keep your application reliable and scalable. It’s not almost spotting failures—it’s about being familiar with your program and ensuring that it works perfectly, even under pressure.
Remaining Ideas
Scalability isn’t only for large corporations. Even smaller apps will need a strong Basis. By designing meticulously, optimizing sensibly, and using the suitable resources, you may Develop applications that mature easily devoid of breaking under pressure. Commence smaller, think massive, and Establish intelligent.